This is Part 1 of a multi-part series on creating a simple iOS application for scuba divers that calculates the Maximum Operating Depth (MOD) of a Nitrox gas mix
Background
Before we dive into the implementation details, let's have a look at what we are going to build and why it can be useful.
Enriched Air Nitrox (EAN), commonly referred to as just Nitrox is a gas mixture of oxygen, nitrogen and a minimal amount of other gasses. The normal Air that we breathe consists of 21% oxygen, 78% nitrogen, and 1% other gasses. EAN, as the name implies, is usually a mix enriched with more oxygen. It's widely used in recreational and technical diving because the reduced amount of nitrogen allows longer bottom time and reduced narcotic effects of the nitrogen at depth. However, like everything else in life, this doesn't come for free and without risks - beyond certain depths, the oxygen in the mix can become toxic and affect the nervous system of the diver and even cause death.
That's why it's very important the gas to be properly analyzed and the Max Operating Depth (MOD) to be calculated and marked on the cylinder.
Requirements
The basic requirements for this calculator are:
- Calculate and display MOD based on selected oxygen percentage
- The oxygen percentage range should be between 21% and 100%
- Allow selection of Partial Pressure of Oxygen (ppO₂) with values 1.4 / 1.5 / 1.6
- Allow selection of metric or imperial measurement units from a settings screen
- Remember the user settings between sessions
along the way, we might add more requirements and enhancements, but for an MVP version these should be enough for us to get started.
Concept
It's always good to have a basic idea of what the application will look like before you start building it, even if it's a few boxes drawn on a napkin.
What we have here is:
- Two tabs - MOD calculator and Settings screen
- Basic text description of what we are calculating
- Slider that changes the oxygen percentage
- ppO₂ selector
- Updating text for EAN mixture and the calculated MOD
Project Setup
At the time of writing the latest version of MacOS is Ventura 13.1, I'm using Xcode 14.2 and targeting iOS 16.2. However, given the simplicity of the app, it should be possible to target even older versions.
Let's start with creating a new project.
Fire up Xocde and select "Create a new Xcode project"
We'll use the basic App template for iOS
for Product Name, we can set NitroxCalculator and for Organization Identifier com.example.dev.nikz but you can switch to whatever you want. The rest of the options remain with the default values
Now let's define the Display Name and change a few more settings:
- Select the project and go to the General tab of the
NitroxCalculator
target - Change Display Name to Nitrox Calculator
- Uncheck Landscape Left and Landscape Right
TabView
Now we can define the basic TabView structure.
For a start, it will be just a placeholder Text and tab icons using Label with systemImage
.
struct ContentView: View {
var body: some View {
TabView {
Text("MOD Calc TODO").tabItem {
Label("MOD", systemImage: "gauge")
}
Text("Settings TODO").tabItem {
Label("Settings", systemImage: "gear")
}
}
}
}
Result
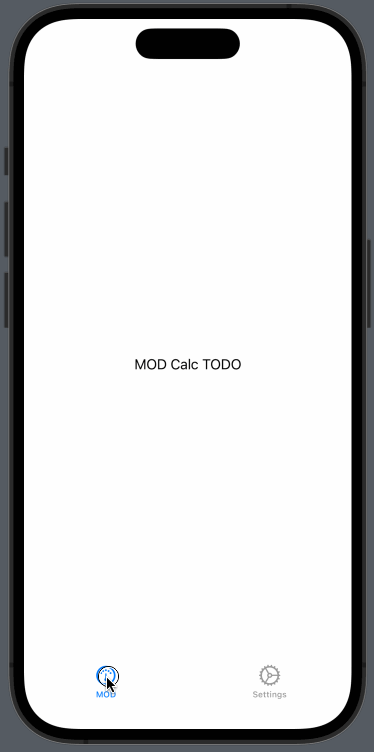
Next
Next, we will implement the settings screen and the basic structure of the MOD calculator screen...